1. Introduction
The FirstNet Call Management API allows a third party application to create and manage calls between mobile phones. The API provides functions to application developers for the following:
- Establish a call session between calling participant and called participant
- Obtain information of a call session
- Terminate a call session
Onboarding Prerequisites:
- The developer must onboard to the AT&T Multi-Services Proxy (MSP) platform.
- The developer’s app must be listed in the App Catalog.
- The developer must onboard to the FirstNet Mobile SSO platform and, for native mobile application development, the developer must also get access to the Mobile SSO libraries.
- The developer of a browser-based application must onboard to the Halo platform.
2. Provisioning & Authorization
Apps utilizing the FirstNet service will need to be provisioned (refer to the Prerequisites section) and will need to supply an OAuth access token with all API requests.
To retrieve an OAuth access token, multiple authentication and authorization methods are supported with Client Credentials and FirstNet SSO credentials (OAuth model - Password Grant).
To get FirstNet SSO credentials (OAuth model - password grant i.e. authorization from an end user) the developer must use the HALO platform in conjunction with the Mobile Key flow for a native app.
2.1 Authorization
The App will first call the OAuth 2.0 Authorization API to get the OAuth access tokens.
The OAuth 2.0 Authorization API provides a highly secure way for AT&T wireless customers to access the AT&T wireless network through a third-party app with less risk of compromising security. This API helps ensure that sensitive AT&T wireless customer-related details are not exposed to the third-party app.
Note: The OAuth 2.0 Authorization API provided by AT&T has the following considerations for you to keep in mind:
- You should specify an API scope in your request that includes all of the APIs that you plan to use.
2.1.1 OAuth Scopes
Each of the APIs in the FirstNet service require specific scopes assigned to the access tokens use in the calls to those APIs. These scopes are as follows:
Model | OAuth Scope Value | Description |
---|---|---|
Client Credential |
FIRSTNET:CALLMGMT |
For supporting third party call management functionality without FirstNet User Authentication. |
Password Grant |
USER:FIRSTNET:CALLMGMT |
For supporting third party call management functionality with FirstNet User Authentication. Note: This uses MobileKey(SSO) for FirstNet user authentication. |
2.1.2 Get Access Token
The purpose of the Get Access Token method is to obtain an OAuth access token that can then be presented by the authorized application to make subsequent requests to AT&T APIs.
The app must provide the App Key and App Secret pair for either the Sandbox or Production instance of the app account in order to obtain an OAuth access token.
The OAuth access token can be obtained in the following ways:
- Using the Password Grant: This mechanism is used directly as an authorization grant to obtain an access token. Instead of a password, this grant type uses a HALO authentication token. This mechanism can only be used if at least one of the requested scopes uses the password grant_type.
- Using the Client Credentials: Using the Client Credentials: This mechanism is used when the application requires no customer authorization and consent capture.
- Using a Refresh Token: The refresh token mechanism can be used to obtain a new OAuth access token. The access token is valid until the time indicated in the expires_in parameter is reached. When a token is about to expire, an application should refresh it using the refresh token. Upon obtaining a new OAuth access token, the original refresh token and OAuth access token are invalidated and can no longer be used.
Note: The refresh token value is not returned for the Password Grant type.
Parameter | Data Type | Req? | Brief description | Location |
---|---|---|---|---|
accept |
String |
No |
Specifies the format of the body of the response. Valid values are:
|
Header |
content-length |
Integer |
No |
Specifies the length of the content in octets. This header parameter is only required for the non-streaming request. |
Header |
content-type |
String |
Yes |
Specifies the type of content of the body of the entity. Must be set to one of the following values:
|
Header |
grant_type |
String |
Yes |
Specifies the mechanism used to obtain the OAuth access token. The following types are supported:
Note: This is not required in the password grant. |
Body |
client_id |
String |
Yes |
Specifies the App Key that is generated after the app has been successfully created. |
Body |
client_secret |
String |
Yes |
Specifies the App Secret that is generated after the app has been successfully created. |
Body |
username |
String |
Cond |
Specifies the access identifier (ID) CTN/Mobile number. |
Body |
password |
String |
Cond |
Specifies the Halo token. |
Body |
refresh_token |
String |
Cond |
Specifies the refresh token value that was returned by a previous Get Access Token method request. Use the refresh_token to obtain a new OAuth access token when the initial OAuth access token expires. |
Body |
scope |
String |
Cond |
Specifies a comma-delimited list of case sensitive strings, where each string defines access to a specific service. The strings are defined by the API Gateway. |
Body |
Parameter | Data Type | Req? | Brief description | Location |
---|---|---|---|---|
content-type |
String |
Yes |
Specifies the type of content of the body of the entity. Only value returned for this parameter is:
|
Header |
access_token |
String |
Yes |
Specifies the OAuth access token issued by the API Gateway. |
Body |
expires_in |
String |
Yes |
Specifies the lifetime, in seconds, of the OAuth access token. For example, the value "3600" denotes that the access token will expire in one hour from the time the response was generated. |
Body |
refresh_token |
String |
Cond |
Specifies the refresh token, that is able to be used to obtain access tokens. Refresh tokens are issued to the client app by the API Gateway and are used to obtain a new OAuth access token when the current OAuth access token becomes non-valid or expires. (This value will not be returned for the Password Grant.) |
Body |
token_type |
String |
Yes |
Specifies the type for the new OAuth access token. The only value returned for this parameter is:
|
Body |
Grant_Type: Client Credentials
Call flow
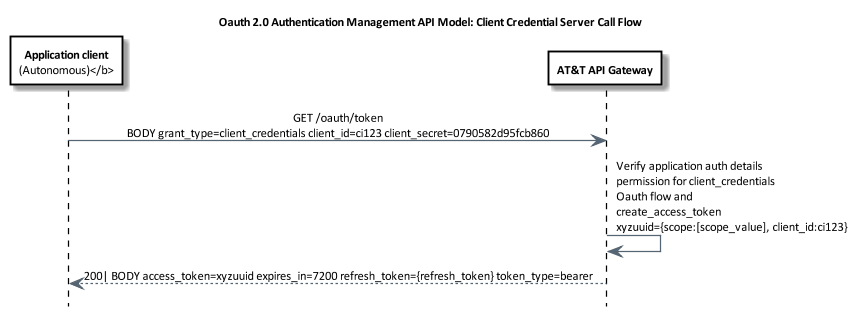
Request - Client credentials grant type
The following example shows how to get an access token using the Password Grant credential. The value of the Accept parameter specifies that the response from the API Gateway should be in JSON.
POST /oauth/v4/token HTTP/1.1 Host: api.att.com Content-Type: application/x-www-form-urlencoded Accept: application/json User-Agent: curl/7.37.0 Content-Length: 162
client_id=ABCDEF0123456789ABCDEF0123456789&client_secret=ABCDEF0123456789&grant_type=client_credentials&scope=FIRSTNET:CALLMGMT
Request - Refresh token (valid only for Client Credentials grant type)
The following example demonstrates how to get an access token based on a previously acquired access token. The value of the Accept parameter specifies that the response from the API Gateway should be in JSON.
POST /oauth/v4/token HTTP/1.1 Host: api.att.com Content-Type: application/x-www-form-urlencoded Accept: application/json User-Agent: curl/7.37.0 Content-Length: 162 client_id=ABCDEF0123456789ABCDEF0123456789&client_secret=ABCDEF012 3456789&grant_type=refresh_token&refresh_token=ABCDEF0123456789ABC DEF0123456789ABCDEF0123456789
Response (Client credentials grant type)
The following example shows a Get Access Token Response in JSON format.
HTTP/1.1 200 OK Content-Type: application/json Cache-Control: no-store Date: Wed, 30 Mar 2011 07:18:40 GMT { "access_token": "b305a68014c81ddd6e9dcf172d2a1064", "expires_in": "7200", "refresh_token": "d6439f42285acc03566709c4eea4fd49bc189b72", "token_type": "bearer"
Grant_Type: FirstNet SSO credentials (OAuth model – Password Grant)
Prerequisites: (refer to section 2 Provisioning and Authorization)
- The application developer has onboarded to the HALO platform and successfully generated a HALO token via the MobileKey SDK.
- The HALO token will work for valid FirstNet user credentials only.
- Once the 3rd party application gets the HALO token, the developer hosted server must use the following API to get the access token.
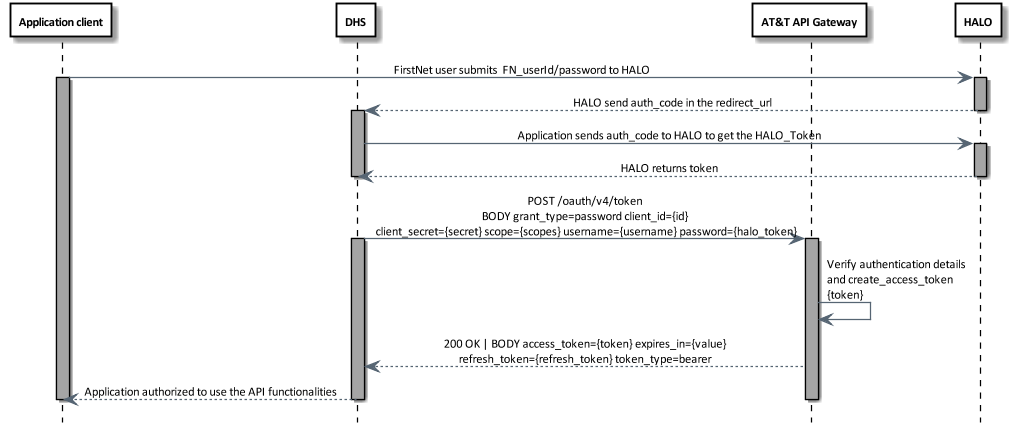
Request - Password Grant
The following example shows how to get an access token using the Password grant. The value of the Accept parameter specifies that the response from the API Gateway should be in JSON.
POST /oauth/v4/token HTTP/1.1 Host: api.att.com Content-Type: application/x-www-form-urlencoded Accept: application/json User-Agent: curl/7.37.0 Content-Length: 162 client_id=ABCDEF0123456789ABCDEF0123456789&client_secret=ABCDEF0123456789&grant_type=password&username={CTN/Mobile Number}&password={HALO_TOKEN}&scope
Response
The following example shows a Get Access Token Response in JSON format.
HTTP/1.1 200 OK Content-Type: application/json Cache-Control: no-store Date: Wed, 30 Mar 2011 07:18:40 GMT { "access_token": "b305a68014c81ddd6e9dcf172d2a1064", "expires_in": "7200", "refresh_token": "d6439f42285acc03566709c4eea4fd49bc189b72", "token_type": "bearer" }
3.1 FirstNet Call Management API
Version | Resource URL relative to | Status |
---|---|---|
1 |
https://api.att.com/firstNetCallManagement/v1 |
Active |
3.1.1 Data Types - Structures
Parameter | Data Type | Req? | Brief description |
---|---|---|---|
participants |
Array of participant object |
Yes |
Specifies participants in this call. The first element in this array denotes the calling participant (originator or source party) of the call session. |
terminated |
Boolean |
No |
Indicates whether the session has been terminated (true) or is active (false). Default value is false. |
mediaInfo |
Array of MediaInfo |
No |
When applicable, it indicates the media currently used in the call for the identified participant. |
link |
Link |
No |
List the Links to other resources that are in relationship with the resource |
clientCorrelator |
String |
No |
Specifies a correlator that the client may use to tag this particular resource representation, during a request to create a resource on the server. Maximum length of field is 256. |
Parameter | Data Type | Req? | Brief description |
---|---|---|---|
participantAddress |
String |
Yes |
Specifies the address of the user. |
participantName |
String |
No |
Specifies name of the participant. |
participantStatus |
CallParticipantStatus Enum |
No |
Indicates the current status of the participant in the call. |
startTime |
Date |
No |
Indicates the time when the participant was added to the call. |
duration |
int |
No |
Indicates the duration of the participant’s involvement in the call, expressed in seconds. |
terminationCause |
CallParticipantTerminationCause Enum |
No |
Indicates the cause of the call participants termination from the call |
mediaInfo |
Array of MediaInfo |
No |
When applicable, it indicates the media currently used in the call for the identified participant. |
link |
Array of Link |
No |
Links to other resources that are in relationship with the resource |
clientCorrelator |
String |
No |
Specifies a correlator that the client may use to tag this particular resource representation, during a request to create a resource on the server. If the field is present, the server does not alter its value and provides it as part of the representation of this resource. If the field is not present, the server generates it. |
CallParticipantTerminationCause Enumeration
Valid Values
CallParticipantNoAnswer
CallParticipantBusy
CallParticipantNotReachable
CallParticipantHangup
CallParticipantAborted
CallParticipantStatus Enumeration
Valid Values
CallParticipantInitial
CallParticipantConnected
CallParticipantTerminated
4.1.1 Initiate Call Session
Functional Behavior
This operation is used to initiate a call session between 2 participants (source party and destination party). Once the API has executed, the source participant’s device (phone) rings. When the source participant answers the phone, the destination participant’s device will ring.
Call Flow
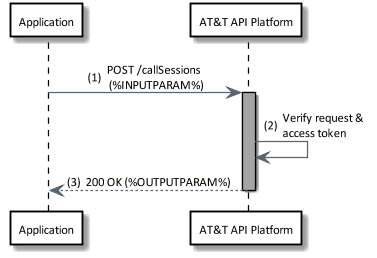
Parameter | Data Type | Req? | Brief description | Location |
---|---|---|---|---|
accept |
String |
No |
Specifies the format of the body of the response. Valid values: application/json |
Header |
authorization |
String |
Yes |
Specifies the authorization type and token. "Bearer" + OAuth Token – access_token. If the authorization header is missing, the system shall return an HTTP 401 Unauthorized message. If the token is invalid the system shall return an HTTP 401 Unauthorized message with a WWW-Authenticate HTTP header. |
Header |
content-length |
integer |
No |
Specifies the length of the content in octets. |
Header |
content-type |
String |
Yes |
Specifies the type of content of the body of the entity. Valid values: application/json |
Header |
callSession |
Object |
Yes |
Specifies the call session information to make a call. |
Body |
Request - Example (Client Credentials)
POST /firstnetCallManagement/v1/callSessions HTTP 1.1 Host: api.att.com Authorization: Bearer Y3BzOmNwczEyMw == Content-Type: application/json Accept: application/json { "callSession": { "clientCorrelator": "123456756", "participant": [ { "participantAddress": "sip:+19876543210@firstnet.com", "participantName": "Source Party" }, { "participantAddress": "sip:+19876543210@firstnet.com", "participantName": "Destination Party" } ] } }
Request - Example (Password Grant)
Note: the first source participantAddress is not required and will be retrieved from the access_token.
POST /firstnetCallManagement/v1/callSessions HTTP 1.1 Host: api.att.com Authorization: Bearer Y3BzOmNwczEyMw == Content-Type: application/json Accept: application/json { "callSession": { "clientCorrelator": "123456756", "participant": [ { "participantName": "Source Party" }, { "participantAddress": "sip:+19876543210@firstnet.com", "participantName": "Destination Party" } ] } }
Output Parameters
Parameter | Data Type | Req? | Brief description | Location |
---|---|---|---|---|
content-type |
String |
Yes |
Specifies the type of content of the body of the entity. Valid values: application/json. |
Header |
content-length |
Integer |
Yes |
The Content-Length entity-header field indicates the size of the entity-body, in decimal number of OCTETs, sent to the recipient or, in the case of the HEAD method, the size of the entity-body that would have been sent had the request been a GET. |
Header |
location |
AnyURI |
Yes |
Specifies the location of the newly created resource |
Header |
callSession |
Object |
Yes |
Specifies the call session information to make a call. |
Body |
Response - Example
HTTP/1.1 201 Created Content-Type: application/json Content-Length: 624 Location: https://api.att.com/firstnetCallManagement/v1/callSessions/callsession1234001 Date: Thu, 04 Jun 2010 02:51:59 GMT { "callSession": { "clientCorrelator": "123456756", "participant": [ { "participantAddress": "sip:+19876543210@firstnet.com", "participantName": "Source Party", "participantStatus": "CallParticipantInitial" }, { "participantAddress": "sip:+19876543210@firstnet.com", "participantName": "Destination Party", "participantStatus": "CallParticipantInitial" } ], "terminated": false } }
Response - HTTP Status Codes
Code | Reason Phrase | Description |
---|---|---|
201 |
Created |
Successful response. The resource was successfully created. |
400 |
Bad Request |
Many possible reasons not specified by the other codes. |
401 |
Unauthorized |
Authentication failed or was not provided. |
403 |
Forbidden |
Access permission error. |
404 |
Not Found |
The server has not found anything matching the Request-URI. No indication is given of whether the condition is temporary or permanent. |
409 |
Conflict |
The request could not be completed due to a conflict with the current state of the resource. This code is only allowed in situations where it is expected that the user might be able to resolve the conflict and resubmit the request. |
413 |
Request Entity Too Large |
The size of the request body exceeded the maximum size permitted. |
415 |
Unsupported Media Type |
The request is in a format not supported by the requested resource for the requested method. |
429 |
Too Many Requests |
The user has sent too many requests in a given amount of time. Intended for use with rate limiting schemes. |
500 |
Internal Server Error |
The server encountered an internal error or timed out; please retry. |
503 |
Service Unavailable |
The server is currently unable to receive requests; please retry. |
Service Exceptions
MessageID | Text | Variables | HTTP Status Codes |
---|---|---|---|
General-0001 |
A service error occurred. Error code is %1 |
%1: Error code from service |
4XX and 5XX error codes |
General-0002 |
Invalid input value for parameter(s) %1 |
%1: parameter |
400 |
General-0003 |
Invalid input value for parameter %1, valid values are %2 |
%1: parameter |
400 |
General-0004 |
Missing mandatory parameter %1 |
%1: Parameter name |
400 |
4.1.2 Get Call Session
Functional Behavior
This operation retrieves the information of an existing call session.
Call flow
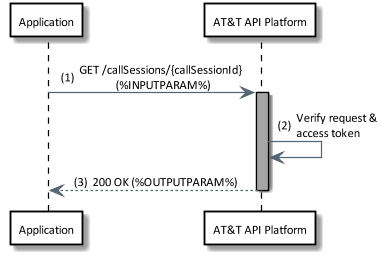
Input Parameters
Parameter | Data Type | Req? | Brief description | Location |
---|---|---|---|---|
accept |
String |
No |
Specifies the format of the body of the response. Valid values are: application/json The default value is application/json. |
Header |
authorization |
String |
Yes |
Specifies the authorization type and token. "Bearer" + OAuth Token – access_token. If the authorization header is missing, the system shall return an HTTP 401 Unauthorized message. If the token is invalid the system shall return an HTTP 401 Unauthorized message with a WWW-Authenticate HTTP header. |
Header |
x-att-msisdn |
String |
Cond. |
Specifies phone number or Subscriber mobile number. Note: This is required for Client Credential grant only. |
Header |
callSessionId |
String |
Yes |
Specifies the identifier of a call session. |
URI Path |
Request - Example (Client credentials grant)
GET /firstNetCallManagement/v1/callSessions/callsession1234001 HTTP/1.1 Host: api.att.com authorization: Bearer abcdef12345678 accept: application/json x-att-msisdn: {phone number}
Request - Example (FirstNet SSO Credentials)
GET /firstNetCallManagement/v1/callSessions/callsession1234001 HTTP/1.1 Host: api.att.com authorization: Bearer abcdef12345678 accept: application/json
Output Parameters
Parameter | Data Type | Req? | Brief description | Location |
---|---|---|---|---|
content-type |
String |
Yes |
Specifies the type of content of the body of the entity. Supported values are: application/json. Note: Even if the expected successful response has no entity body, this parameter could still be present in an error message, where the error message has an entity body. |
Header |
callSession |
Object |
Yes |
Specifies the container object for the call session |
Body |
Response - Example
HTTP/1.1 200 Ok Content-Type: application/json Content-Length: 624 Date: Thu, 04 Jun 2010 02:51:59 GMT { "callSession": { "clientCorrelator": "123456756", "participant": [ { "participantAddress": "sip:+19876543210@firstnet.att.net.com", "participantName": "Source Party", "participantStatus": "CallParticipantInitial" }, { "participantAddress": "sip:+19876543210@firstnet.att.net.com", "participantName": "Destination Party", "participantStatus": "CallParticipantInitial" } ], "terminated": false }
Response - HTTP Status Codes
Code | Reason Phrase | Description |
---|---|---|
200 |
OK |
Successful response. |
400 |
Bad Request |
Many possible reasons not specified by the other codes. |
401 |
Unauthorized |
Authentication failed or was not provided. |
403 |
Forbidden |
Access permission error. |
404 |
Not Found |
The server has not found anything matching the Request-URI. No indication is given of whether the condition is temporary or permanent. |
405 |
Method Not Allowed |
A request was made of a resource using a request method not supported by that resource, for example using PUT on a REST resource that only supports POST. |
410 |
Gone |
Indicates that the resource requested is no longer available and will not be available again. This should be used when a resource has been intentionally removed and the resource should be purged. |
429 |
Too Many |
The user has sent too many requests in a given amount of time. Intended for use with rate limiting schemes. |
500 |
Internal Server Error |
The server encountered an internal error or timed out; please retry. |
503 |
Service Unavailable |
The server is currently unable to receive requests; please retry. |
Service Exceptions
MessageId | Text | Variables | HTTP Status Code |
---|---|---|---|
General-0001 |
A service error occurred. Error code is %1 |
%1: Error code from service |
4XX and 5XX error codes |
General-0002 |
Invalid input value for parameter(s) %1 |
%1: parameter |
400 |
General-0003 |
Invalid input value for parameter %1, valid values are %2 |
%1: parameter |
400 |
General-0004 |
Missing mandatory parameter %1 |
%1: parameter |
400 |
4.1.3 Terminate Call Session
Functional Behavior
This operation terminates an existing call session.
Call flow
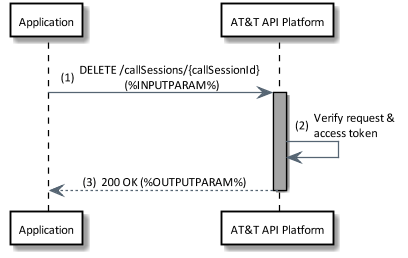
Input Parameters
Parameter | Data Type | Req? | Brief description | Location |
---|---|---|---|---|
accept |
String |
No |
Specifies the format of the body of the response. Valid values are: application/json The default value is application/json. |
Header |
authorization |
String |
Yes |
Specifies the authorization type and token. "Bearer" + OAuth Token – access_token. If the authorization header is missing, the system shall return an HTTP 401 Unauthorized message. If the token is invalid the system shall return an HTTP 401 Unauthorized message with a WWW-Authenticate HTTP header. |
Header |
x-att-msisdn |
String |
Cond. |
Specifies phone number or Subscriber mobile number. Note: This is required for Client Credential grant only. |
Header |
callSessionId |
String |
Yes |
Specifies the identifier of a call session. |
URI Path |
Request - Example (Client Credentials)
DELETE /firstNetCallManagement/v1/callSessions/callsession1234001 HTTP/1.1 Host: api.att.com authorization: Bearer abcdef12345678 accept: application/json x-att-msisdn: {phone number}
Request - Example (FirstNet SSO Credentials)
DELETE /firstNetCallManagement/v1/callSessions/callsession1234001 HTTP/1.1 Host: api.att.com authorization: Bearer abcdef12345678 accept: application/json
Output Parameters
Parameter | Data Type | Req? | Brief description | Location |
---|---|---|---|---|
content-type |
String |
Yes |
Specifies the type of content of the body of the entity. Supported values are: application/json. |
Header |
content-length |
Integer |
Yes |
The Content-Length entity-header field indicates the size of the entity-body, in decimal number of OCTETs, sent to the recipient or, in the case of the HEAD method, the size of the entity-body that would have been sent had the request been a GET. |
Header |
callSession |
Object |
Yes |
Specifies the container object for the call session |
Body |
Response - Example
HTTP/1.1 200 Ok Content-Type: application/json Content-Length: 624 Date: Thu, 04 Jun 2010 02:51:59 GMT { "callSession": { "clientCorrelator": "123456756", "participant": [ { "duration" : 30, "participantAddress": "sip:+19876543210@firstnet.att.net.com", "participantName": "Source Party", "participantStatus": "CallParticipantInitial", "startTime" : "2018-11-01T18:39:09.267Z", "terminationCause" : "CallParticipantAborted" }, { "duration" : 28, "participantAddress": "sip:+19876543210@firstnet.att.net.com", "participantName": "Destination Party", "participantStatus": "CallParticipantInitial", "startTime" : "2018-11-01T18:39:09.267Z", "terminationCause" : "CallParticipantAborted" } ], "terminated": "true" }
Response - HTTP Status Codes
Code | Reason Phrase | Description |
---|---|---|
200 |
OK |
Successful response |
400 |
Bad Request |
Many possible reasons not specified by the other codes. |
401 |
Unauthorized |
Authentication failed or was not provided. |
403 |
Forbidden |
Access permission error. |
404 |
Not Found |
The server has not found anything matching the Request-URI. No indication is given of whether the condition is temporary or permanent. |
405 |
Method Not Allowed |
A request was made of a resource using a request method not supported by that resource, for example using PUT on a REST resource that only supports POST. |
429 |
Too Many Requests |
The user has sent too many requests in a given amount of time. Intended for use with rate limiting schemes. |
500 |
Internal Server Error |
The server encountered an internal error or timed out; please retry. |
503 |
Service Unavailable |
The server is currently unable to receive requests; please retry. |
Service Exceptions
MessageId | Text | Variables | HTTP Status Code |
---|---|---|---|
General-0001 |
A service error occurred. Error code is %1 |
%1: Error code from service |
4XX and 5XX error codes |
General-0002 |
Invalid input value for parameter(s) %1 |
%1: parameter |
400 |
General-0003 |
Invalid input value for parameter %1, valid values are %2 |
%1: parameter |
400 |
General-0004 |
Missing mandatory parameter %1 |
%1: parameter |
400 |
5 Structure of Exceptions
RESTful Webservices Exceptions
Two types of RESTful exceptions are supported:
- Service Exceptions: These exceptions occur when a service is unable to process a request and retrying the request will result in a consistent failure. For example, if an application provides invalid input.
- Policy Exceptions: These exceptions occur when a policy criterion has not been met. For example, if (N+1)th request arrives when an application's service level agreement only allows for N transactions per second.
The error information will be in the entity body as well as the entity body. This serves as a migration from having the errors in the body to the errors in the header, so both will be supported until a date is determined to discontinue the errors in the body.
Both service and policy exceptions contain the following elements:
Field Name | Data Type | Req? | Description | Location |
---|---|---|---|---|
x-att-errorInfo |
xs:string |
Yes |
A link to the documentation regarding this error. Application Developers can follow the link to get additional information regarding the meaning, likely causes and solutons of this error. The URI should take the form of: http://developer.att.com/apis/error-detail?error_code=<error_code> |
Header |
x-att-errorMessageId |
xs:string |
Yes |
Unique message identifier of the format ‘ABCnnnn’ where ‘ABC’ is either ‘SVC’ for Service Exceptions or ‘POL’ for Policy Exception. |
Header |
x-att-errorText |
xs:string |
Yes |
Message text, with replacement variables marked with %n, where n is an index into the list of <variables> elements, starting at 1. |
Header |
x-att-errorVariables |
xs:string |
Yes |
List of zero or more strings that represent the contents of the variables used by the message text. |
Header |
x-att-errorType |
xs:string |
Yes |
The type of error, either service or policy |
Header |
MessageId |
xs:string |
Yes |
Unique message identifier of the format ‘ABCnnnn’ where ‘ABC’ is either ‘SVC’ for Service Exceptions or ‘POL’ for Policy Exception. |
Body |
Text |
xs:string |
Yes |
Message text, with replacement variables marked with %n, where n is an index into the list of <variables> elements, starting at 1. |
Body |
Variables |
xs:string [0..unbounded] |
No |
List of zero or more strings that represent the contents of the variables used by the message text. |
Body |
Sample exception responses are provided below:
SVC0001 Exception - JSON Body:
HTTP/1.1 400 Bad Request Content-Type: application/json Content-Length: 115 Date: Thu, 04 Jun 2014 02:51:59 GMT x-att-errormessageId: SVC0001 x-att-errortext: A service error occurred. Error code is %1 x-att-errorvariables: error message from system x-att-errorType: service x-att-errorInfo: http://developer.att.com/apis/error-detail?error_code=SVC0001 { "requestError": { "serviceException": { "messageId": "SVC0001", "text": "A service error occurred. Error code is %1", "variables": "error message from system" } } }
SVC0002 Exception - JSON Body:
HTTP/1.1 400 Bad Request Content-Type: application/json Content-Length: 115 Date: Thu, 04 Jun 2014 02:51:59 GMT x-att-errormessageId: SVC0002 x-att-errortext: Invalid input value for message part %1 x-att-errorvariables: count x-att-errorType: service x-att-errorInfo: http://developer.att.com/apis/error-detail?error_code=SVC0002 { "requestError": { "serviceException": { "messageId": "SVC0002", "text": "Invalid input value for message part %1", "variables": "count" } } }
SVC0003 Exception - JSON Body:
HTTP/1.1 400 Bad Request Content-Type: application/json Content-Length: 11SVC0001 Date: Thu, 04 Jun 2014 02:51:59 GMT x-att-errormessageId: SVC0003 x-att-errortext: Invalid input value for message part %1, valid values are %2 x-att-errorvariables: size,small,medium,large x-att-errorType: service x-att-errorInfo: http://developer.att.com/apis/error-detail?error_code=SVC0003 { "requestError": { "serviceException": { "messageId": "SVC0003", "text": "Invalid input value for message part %1, valid values are %2", "variables": "size,small,medium,large" } } }
SVC1002 Exception - JSON Body:
HTTP/1.1 400 Bad Request Content-Type: application/json Content-Length: 115 Date: Thu, 04 Jun 2014 02:51:59 GMT x-att-errormessageId: SVC1002 x-att-errortext: Missing mandatory parameter %1 x-att-errorvariables: count x-att-errorType: service x-att-errorInfo: http://developer.att.com/apis/error-detail?error_code=SVC1002 { "requestError": { "serviceException": { "messageId": "SVC1002", "text": "Missing mandatory parameter %1", "variables": "count" } } }
SVC1002 Exception (When Authorization parameter is missing) - JSON Body:
HTTP/1.1 401 Unauthorized Content-Type: application/json Content-Length: 115 Date: Thu, 04 Jun 2014 02:51:59 GMT x-att-errormessageId: SVC1002 x-att-errortext: Missing mandatory parameter %1 x-att-errorvariables: authorization x-att-errorType: service x-att-errorInfo: http://developer.att.com/apis/error-detail?error_code=SVC1002 { "requestError": { "serviceException": { "messageId": "SVC1002", "text": "Missing mandatory parameter %1", "variables": "authorization" } } }
URI Location Not Found (Due to a parameter as an URI path element)
HTTP/1.1 404 Not Found Content-Type: application/json Content-Length: 186 Date: Thu, 04 Jun 2014 02:51:59 GMT x-att-errormessageId: SVC1002 x-att-errortext: Missing mandatory parameter %1 x-att-errorvariables: someId x-att-errorType: service x-att-errorInfo: http://developer.att.com/apis/error-detail?error_code=SVC1002 { "requestError": { "serviceException": { "messageId": "SVC1002", "text": "Missing mandatory parameter %1", "variables": "someId" } } }
POL0001 Exception - JSON Body:
HTTP/1.1 401 Unauthorized Content-Type: application/json Content-Length: 115 Date: Thu, 04 Jun 2014 02:51:59 GMT x-att-errormessageId: POL0001 x-att-errortext: A policy error occurred. Error code is %1 x-att-errorvariables: invalid accesstoken x-att-errorType: policy x-att-errorInfo: http://developer.att.com/apis/error-detail?error_code=POL0001 { "requestError": { "PolicyException": { "messageId": "POL0001", "text": "A policy error occurred. Error code is %1", "variables": "invalid accesstoken" } } }
HTTP 503 - with retry:
There may be situations when the server becomes overloaded due to aggregated TPS limits being reached. In this can a HTTP 503 will be returned with a Retry-After HTTP header giving the number of seconds to wait to retry the request.
HTTP/1.0 503 Service Unavailable Retry-After: 60 Content-Length: 0 Date: Thu, 04 Jun 2014 02:51:59 GMT x-att-errormessageId: x-att-errortext: x-att-errorvariables: x-att-errorType: x-att-errorInfo: http://developer.att.com/apis/error-detail?error_code=http503
HTTP 503 - without retry:
HTTP/1.0 503 Service Unavailable Content-Length: 0 Date: Thu, 04 Jun 2014 02:51:59 GMT x-att-errormessageId: x-att-errortext: x-att-errorvariables: x-att-errorType: x-att-errorInfo: http://developer.att.com/apis/error-detail?error_code=http503
Access Token Value Missing or Invalid
This can be due to 2 different scenarios, either the value is completely missing (including the keyword Bearer), or only the token value is missing:
PUT /myservice/v1/items/myitem HTTP/1.1 Host: api.att.com authorization:{message body} PUT /myservice/v1/items/myitem HTTP/1.1 Host: api.att.com authorization: Bearer {message body} Error Message due to the above 2 scenarios: HTTP/1.1 401 Unauthorized Content-Type: application/json Content-Length: 201 Date: Thu, 04 Jun 2014 02:51:59 GMT x-att-errorMessageId: POL0001 x-att-errorText: A policy error occurred. Error code is %1 x-att-errorVariables: invalid accesstoken x-att-errorType: policy x-att-errorInfo: http://developer.att.com/apis/error-detail?error_code=POL0001 { "requestError": { "policyException": { "messageId": "POL0001", "text": "A policy error occurred. Error code is %1", "variables": ["invalid accesstoken"] } } }
Missing Authorization Parameter in Header
HTTP/1.1 401 Unauthorized Content-Type: application/json Content-Length: 194 Date: Thu, 04 Jun 2014 02:51:59 GMT x-att-errorMessageId: SVC1002 x-att-errorText: Missing mandatory parameter %1 x-att-errorVariables: authorization x-att-errorType: service x-att-errorInfo: http://developer.att.com/apis/error-detail?error_code=SVC1002 { "requestError": { "serviceException": { "messageId": "SVC1002", "text": "Missing mandatory parameter %1", "variables": ["authorization"] } } }
Access Token Expired
Access tokens have an expiry time, they can only be used within a certain time duration. If a token is used in a request after the expiry time of the access token, then this error shall be thrown.
HTTP/1.1 419 Authentication Timeout Content-Type: application/json Content-Length: 195 Date: Thu, 04 Jun 2014 02:51:59 GMT x-att-errorMessageId: POL0001 x-att-errorText: A policy error occurred. Error code is %1 x-att-errorVariables: invalid accesstoken x-att-errorType: policy x-att-errorInfo: http://developer.att.com/apis/error-detail?error_code=POL0001 { "requestError": { "policyException": { "messageId": "POL0001", "text": "A policy error occurred. Error code is %1", "variables": ["invalid accesstoken"] } } }
Invalid Scope
If a scope value that is associated with an access token is not the correct scope, then this error shall be thrown.
HTTP/1.1 403 Forbidden Content-Type: application/json Content-Length: 189 Date: Thu, 04 Jun 2014 02:51:59 GMT x-att-errorMessageId: POL0001 x-att-errorText: A policy error occurred. Error code is %1 x-att-errorVariables: invalid scope x-att-errorType: policy x-att-errorInfo: http://developer.att.com/apis/error-detail?error_code=POL0001 { "requestError": { "policyException": { "messageId": "POL0001", "text": "A policy error occurred. Error code is %1", "variables": ["invalid scope"] } } }
Exceeded Rate Limit
Each client is designated with a certain rate limit. If the number of requests exceed the maximum allowed within a time period (i.e. seconds, hours, etc), then this error shall be thrown.
HTTP/1.1 429 Too Many Requests Content-Type: application/json Content-Length: 189 Date: Thu, 04 Jun 2014 02:51:59 GMT x-att-errorMessageId: POL0001 x-att-errorText: A policy error occurred. Error code is %1 x-att-errorVariables: TPS Limit Exceeded x-att-errorType: policy x-att-errorInfo: http://developer.att.com/apis/error-detail?error_code=POL0001 { "requestError": { "policyException": { "messageId": "POL0001", "text": "A policy error occurred. Error code is %1", "variables": ["TPS Limit Exceeded"] } } }